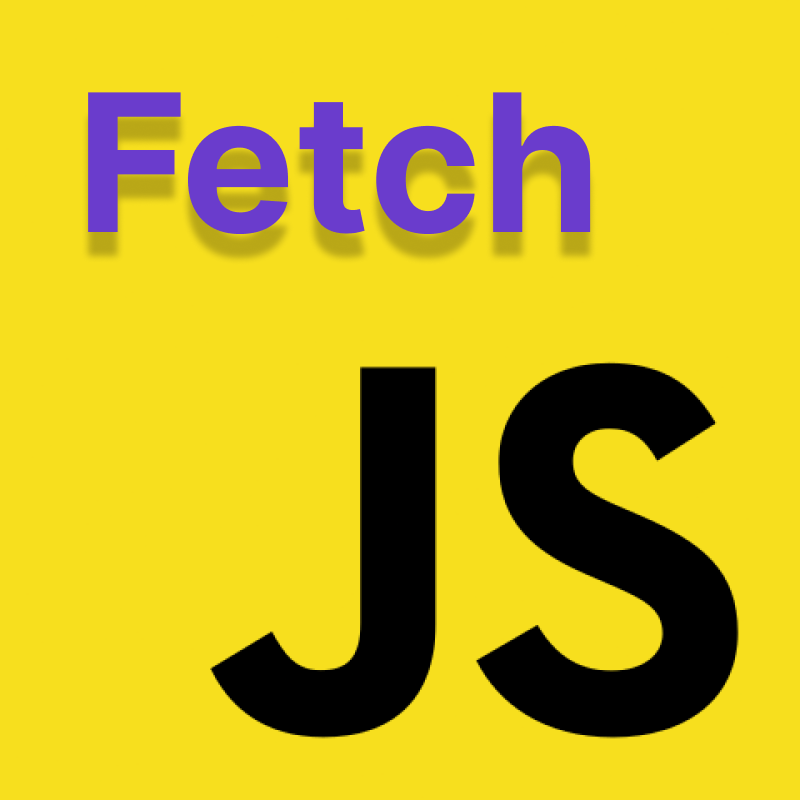
Guides for fetch API
The Fetch API is a browser API that allows you to make network requests similar to XMLHttpRequest (XHR). Unlike XHR, the Fetch API uses Promises, making it easier to handle responses and errors. This guide will walk you through the basics of using the Fetch API to make HTTP requests in JavaScript.
- The first step is to create a new request. You can do this by calling the
fetch()
function and passing it the URL of the resource you want to request. For example, to request a JSON file, you can use the following code:
fetch('https://jsonplaceholder.typicode.com/posts')
- The
fetch()
function returns a promise that resolves to aResponse
object. The response object contains the data returned by the server, as well as information about the status of the request. You can use thejson()
method to parse the response data as JSON.
fetch('https://jsonplaceholder.typicode.com/posts')
.then((response) => response.json())
.then((data) => console.log(data))
- You can also handle errors by using the
catch()
method on the promise.
fetch('https://jsonplaceholder.typicode.com/posts')
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error))
- You can also send data to the server by passing an options object as the second parameter to the
fetch()
function. This object can contain different options likemethod
,headers
,body
, etc. For example, to send a POST request with JSON data, you can use the following code:
fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
title: 'foo',
body: 'bar',
userId: 1,
}),
})
.then((response) => response.json())
.then((data) => console.log(data))
- You can also use async/await instead of Promises. The following example is the equivalent of the previous example, but uses async/await:
async function postData() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
title: 'foo',
body: 'bar',
userId: 1,
}),
})
const data = await response.json()
console.log(data)
} catch (error) {
console.error(error)
}
}
postData()
In this example, we are using the async
keyword to define an asynchronous function, postData()
. Within this function, we are using the await
keyword to wait for the fetch()
function to return a response. The response is then parsed as JSON using the response.json()
method. If there is an error, it will be caught by the catch
block, and the error message will be logged to the console.
In conclusion, the Fetch API is a modern and easy-to-use way to make HTTP requests in JavaScript. It uses Promises to handle responses and errors, and it can be used to retrieve and send data to servers. With basic knowledge of JavaScript, you can use the Fetch API to make network requests and handle their responses.
References: