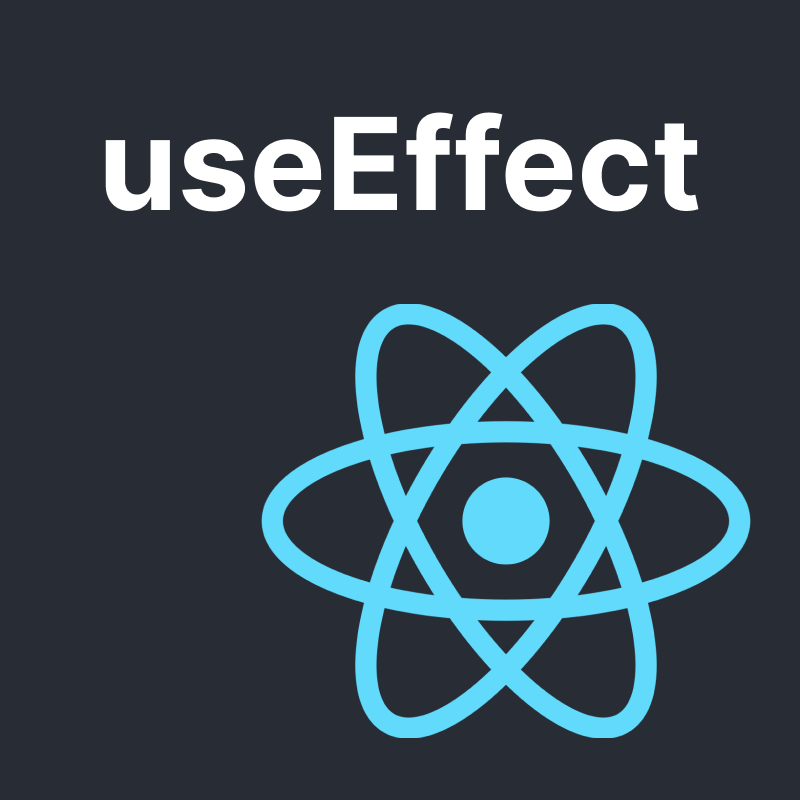
React hook `useEffect` pitfalls
Most common pitfalls of useEffect hook
The useEffect
hook in React is a powerful tool for managing component side effects, such as fetching data, subscribing to events, or updating the DOM. However, there are a few pitfalls to be aware of when using useEffect.
- Infinite Loops: One common pitfall is creating an infinite loop by including a state variable in the dependency array that is updated within the effect. This will cause the effect to run again and again, leading to an infinite loop.
const [count, setCount] = useState(0)
useEffect(() => {
setCount(count + 1)
}, [count]) // This will cause an infinite loop
- Excessive Re-renders: If an effect has a dependency that is not used within the effect, it can cause unnecessary re-renders of the component. This can lead to poor performance, as well as unexpected behavior.
const [name, setName] = useState('John')
const [age, setAge] = useState(25)
useEffect(() => {
console.log('Name changed: ', name)
}, [name, age]) // age is not used in the effect, so it will cause unnecessary re-renders
- Forgetting to Clean Up: If an effect is creating a side-effect, such as a subscription, it is important to clean up the side-effect before the component is unmounted. Failing to do so can lead to memory leaks and other bugs.
useEffect(() => {
const interval = setInterval(() => {
console.log('Tick')
}, 1000)
// missing cleanup function to clearInterval
}, [])
- Incorrect Dependency Array: If the dependency array is not used correctly, it can cause the effect to run more or less often than intended. This can lead to unexpected behavior, such as updating the wrong state, or not updating at all.
const [name, setName] = useState('John')
const [age, setAge] = useState(25)
useEffect(() => {
console.log('Name changed: ', name)
}, [age]) // age is not related to the effect, this will cause unexpected behavior
- Multiple unnecessary useEffect: If multiple useEffect hooks are added for a single component it can lead to unnecessary re-renders and performance issues.
useEffect(() => {
console.log('First useEffect')
}, [data])
useEffect(() => {
console.log('Second useEffect')
}, [data])
useEffect(() => {
console.log('Third useEffect')
}, [data])
- Updating State inside useEffect: If state updates inside useEffect, it may lead to unexpected behavior, because the component will not re-render synchronously and may lead to inconsistent state.
const [count, setCount] = useState(0)
useEffect(() => {
setCount(count + 1)
}, [])
instead do this:
const [count, setCount] = useState(0)
useEffect(() => {
setCount((count) => count + 1)
}, [])
In conclusion, the useEffect
hook is a powerful tool for managing component side effects in React, but it’s important to be aware of the pitfalls that can arise when using it. To avoid infinite loops, too many re-renders, and memory leaks, it’s important to use the useCallback
, useMemo
, and return a cleanup function, when necessary. It’s also important to thoroughly test your code, using tools like the React Dev Tools, to catch and debug any issues that may arise.