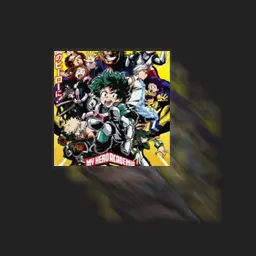
Smooth element transition between pages
Element transition between pages with Vue
Adding animation on specific elements from one page to another can add a smooth transition experience for user. Using vue-route, this can be easily done by passing position and size of an element from previous page, using these params I can animate the elements like this:
Jump right to the code
/* AnimeListItem.vue */
// template
<div class="list__item" @click="onClick" ref="item">
<figure class="image is-square">
<img :src="anime.image_url" :alt="anime.title" />
</figure>
</div>
// methods
methods: {
onClick() {
// getting its position and size
const bound = this.$refs.item.getBoundingClientRect();
// passing bound values to the next page
this.$router.push({
name: 'AnimeDetailPage',
params: { bound }
});
},
},
Now that we have passed the bound
values to the next page, here’s when we create animation on the next page using this param:
(I’m using gsap to help with animation)
/* AnimeDetailPage.vue */
// template
<figure class="image anime__cover" ref="cover">
<img :src="anime.image_url" />
</figure>
mounted() {
const currentBound = this.$refs.cover.getBoundingClientRect();
const previousBound = this.$route.params.bound;
// run animation
gsap.from(this.$refs.cover, {
x: previousBound.x - currentBound.x,
y: previousBound.y - currentBound.y,
width: previousBound.width,
height: previousBound.height,
duration: 0.8,
ease: "power3.out",
});
},
You can checkout the source code here!