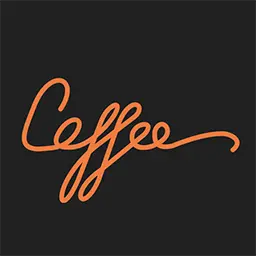
Drawing animation with SVG
I’m sure you’ve seen one of those images or texts with cool animations that looks like it’s drawing itself. There are already many tutorials showing step-by-step on how it is done, and I have little to add, however I think I would explain it one more time how I understand it.
Create an SVG Path
First what you need is an SVG path you want the drawing animation to run with. There are many SVG path generators online, for this example I used SVG Path Builder by Anthony Dugois.
<svg viewBox="0 0 800 600" xmlns="http://www.w3.org/2000/svg" width="600">
<path
id="coffee-path"
d="M 119 273 C 171 194 94 164 53 263 C 32 318 2 374 64 374 C 103 371 134 372 160 331 C 184 288 136 289 112 337 Q 94 375 152 369 Q 188 364 232 318 C 296 245 244 250 223 301 L 164 451 C 110 574 91 460 211 365 L 304 294 C 341 267 298 231 266 309 L 215 453 C 174 544 135 486 221 427 L 331 337 C 368 296 289 307 301 353 Q 310 378 350 367 C 375 357 396 341 388 320 C 380 306 365 309 358 328 Q 351 378 402 366 Q 479 335 529 335 C 554 336 582 355 555 376 "
stroke-width="12"
stroke="#000000"
fill="none"
/>
</svg>
Set stroke pattern
The stroke on the <path>
tag can be set to a dash pattern with attribute stroke-dasharray
. So for example, if I set it to a random number, this is how it looks like:
<svg viewBox="0 0 800 600" xmlns="http://www.w3.org/2000/svg" width="600">
<path
id="coffee-path"
d="M 119 273 C 171 194 94 164 53 263 C 32 318 2 374 64 374 C 103 371 134 372 160 331 C 184 288 136 289 112 337 Q 94 375 152 369 Q 188 364 232 318 C 296 245 244 250 223 301 L 164 451 C 110 574 91 460 211 365 L 304 294 C 341 267 298 231 266 309 L 215 453 C 174 544 135 486 221 427 L 331 337 C 368 296 289 307 301 353 Q 310 378 350 367 C 375 357 396 341 388 320 C 380 306 365 309 358 328 Q 351 378 402 366 Q 479 335 529 335 C 554 336 582 355 555 376 "
stroke-dasharray="10"
stroke-width="12"
stroke="#000000"
fill="none"
/>
</svg>
And we can adjust the length of those dashes:
<svg viewBox="0 0 800 600" xmlns="http://www.w3.org/2000/svg" width="600">
<path
id="coffee-path"
d="M 119 273 C 171 194 94 164 53 263 C 32 318 2 374 64 374 C 103 371 134 372 160 331 C 184 288 136 289 112 337 Q 94 375 152 369 Q 188 364 232 318 C 296 245 244 250 223 301 L 164 451 C 110 574 91 460 211 365 L 304 294 C 341 267 298 231 266 309 L 215 453 C 174 544 135 486 221 427 L 331 337 C 368 296 289 307 301 353 Q 310 378 350 367 C 375 357 396 341 388 320 C 380 306 365 309 358 328 Q 351 378 402 366 Q 479 335 529 335 C 554 336 582 355 555 376 "
stroke-dasharray="100"
stroke-width="12"
stroke="#000000"
fill="none"
/>
</svg>
You see where I’m going with this, now if I make the dash length so long that it cover the entire path, it will appears as if it’s a single line drawn. You can try put a large number like 10000
or in my case I use JS to help to get the exact length of the path:
let path = document.querySelector('#coffee-path')
let pathLength = path.getTotalLength() // exact length of the SVG path
// setting the stroke dash length to cover the entire path
path.setAttribute('stroke-dasharray', pathLength)
Adjust stroke pattern offset
We can adjust the offset of where the stroke pattern dashes should start using attribute stroke-dashoffset
. We will shift it off the path so that it start with empty stroke (you can try with any random number to help visualize):
// adjust offset of dash where it should start
path.setAttribute('stroke-dashoffset', pathLength)
Animate stroke offset back to 0
Finally, to make the drawing animation, we simply adjust the offset slowly back to 0. I use GSAP, but you can use any animation library or just CSS transition also works.
// animate the dash from the shifted offset back to 0
let drawingTl = gsap.timeline()
drawingTl.to(path, {
delay: 0.3,
duration: 5,
'stroke-dashoffset': 0,
ease: 'sine.inOut',
})
That’s it! I hope you find this short article useful.
See the Pen Drawing animation with SVG Path by Aphiwad Chhoeun (@aphiwadchhoeun) on CodePen.